Introduction to Python
Python is a versatile and powerful programming language that has gained immense popularity across the globe. Known for its readability and simplicity, Python is widely used in various fields, including web development, data science, machine learning, and automation. This guide aims to provide a comprehensive overview of Python, highlighting its key features and demonstrating why it is a language worth learning.
Python’s robust community and extensive libraries make it an ideal choice for beginners and experienced programmers alike. Its clean syntax allows developers to focus on solving problems rather than getting bogged down by complex code. Whether you’re building a web application, analyzing data, or developing software, learning Python can open doors to numerous opportunities.
Why Learn Python?
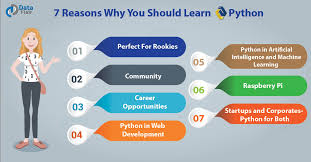
Python has become a popular choice for developers due to its flexibility and ease of use. Here are a few reasons why you should learn Python:
- Wide Range of Applications: Python can be used for web development, data analysis, machine learning, scientific computing, and more.
- Easy to Learn: Python’s simple and intuitive syntax makes it an excellent language for beginners.
- Strong Community Support: Python has a large and active community that provides support, tutorials, and resources.
- Extensive Libraries and Frameworks: Python offers a wealth of libraries and frameworks, such as NumPy, Pandas, Django, and Flask, that simplify development.
- High Demand in the Job Market: Python is one of the most in-demand programming languages, with many job opportunities in various industries.
Python’s versatility also extends to its adaptability in integrating with other languages and technologies. Its cross-platform compatibility means that Python code can run seamlessly on various operating systems, including Windows, macOS, and Linux. This flexibility makes it an ideal choice for developing cross-platform applications.
Moreover, Python’s robust testing frameworks, such as PyTest and Unittest, facilitate efficient debugging and testing processes, ensuring the reliability of software applications. As the tech industry continues to evolve, Python remains a leading choice for innovation, empowering developers to tackle complex problems with ease and creativity.
Setting Up Your Python Environment
Before you start coding, you need to set up your Python environment. Follow these steps to get started:
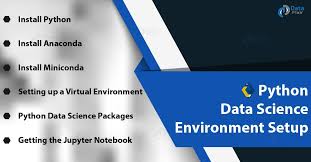
- Download Python: Visit the official Python website to download the latest version of Python for your operating system.
- Install an IDE: Integrated Development Environments (IDEs) like PyCharm, VS Code, and Jupyter Notebook provide a convenient platform for writing and testing Python code.
- Install Python Packages: Use Python’s package manager, pip, to install additional libraries and tools that you might need for your projects.
Understanding Python Syntax
Python’s syntax is designed to be clean and readable, which makes it easy to learn and understand. Here are some key aspects of Python syntax:
- Indentation: Python uses indentation to define code blocks, such as loops and functions.
- Comments: Use the # symbol to add comments to your code, which the Python interpreter ignores.
- Variables: Variables do not require explicit declaration; assign a value to a variable name to create it.
- Statements: Python statements are typically written one per line, and they are executed sequentially.
Python Basics: Data Types and Variables
Python supports a variety of data types, which are used to store and manipulate data. Here are some of the most common data types in Python:
- Integers: Whole numbers without a decimal point (e.g., 10, -5).
- Floats: Numbers with a decimal point (e.g., 3.14, -0.01).
- Strings: Sequences of characters enclosed in quotes (e.g., “Hello, World!”).
- Booleans: Represents truth values, either True or False.
Variables are used to store data in Python. You can assign a value to a variable using the = operator, and you can change the value of a variable at any time.
Python Control Structures
Control structures are used to control the flow of a program. Python offers several control structures, including if statements and loops.
If Statements
If statements are used to execute a block of code if a condition is true. The basic syntax is as follows:
if condition:
# Code to execute if condition is true
Loops
Loops allow you to execute a block of code multiple times. Python supports two types of loops: for loops and while loops.
- For Loops: Iterate over a sequence of elements, such as a list or a string.
- While Loops: Continue executing a block of code as long as a condition is true.
Functions in Python
Functions are reusable blocks of code that perform a specific task. They help organize code and make it more manageable. To define a function in Python, use the def keyword followed by the function name and a pair of parentheses:
def function_name(parameters):
# Code to execute
return value
Functions can take parameters as input and return a value as output. They are essential for creating modular and maintainable code.
Working with Python Collections
Python offers several built-in collection types that allow you to store and manipulate groups of data. Here are some of the most commonly used collections:
Lists
Lists are ordered, mutable collections of items. They are defined using square brackets:
my_list = [1, 2, 3, 4, 5]
Tuples
Tuples are ordered, immutable collections of items. They are defined using parentheses:
my_tuple = (1, 2, 3, 4, 5)
Dictionaries
Dictionaries are collections of key-value pairs. They are defined using curly braces:
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'}
Dictionaries are useful for storing and accessing data using unique keys.
Error Handling: Python Try Except
Python’s try and except mechanism not only helps in gracefully handling known exceptions but also allows for catching multiple types of exceptions using multiple except blocks. This feature enables developers to address different error conditions specifically.
For example, you can handle FileNotFoundError for missing files and ValueError for invalid data separately, providing more precise error messages to users.
try:
# Code that may raise an exception
result = 10 / 0
except ZeroDivisionError:
# Code to execute if an exception is raised
print("Error: Division by zero is not allowed.")
The try block contains the code that may raise an exception, while the except block contains the code to execute if an exception is raised.
Additionally, the final block can be used alongside try and except to ensure that specific cleanup code is executed regardless of whether an exception is raised, such as closing a file or releasing a network resource. This structured approach to error handling enhances code robustness and ensures that applications can continue functioning smoothly even when unexpected issues arise.
Advanced Python Concepts
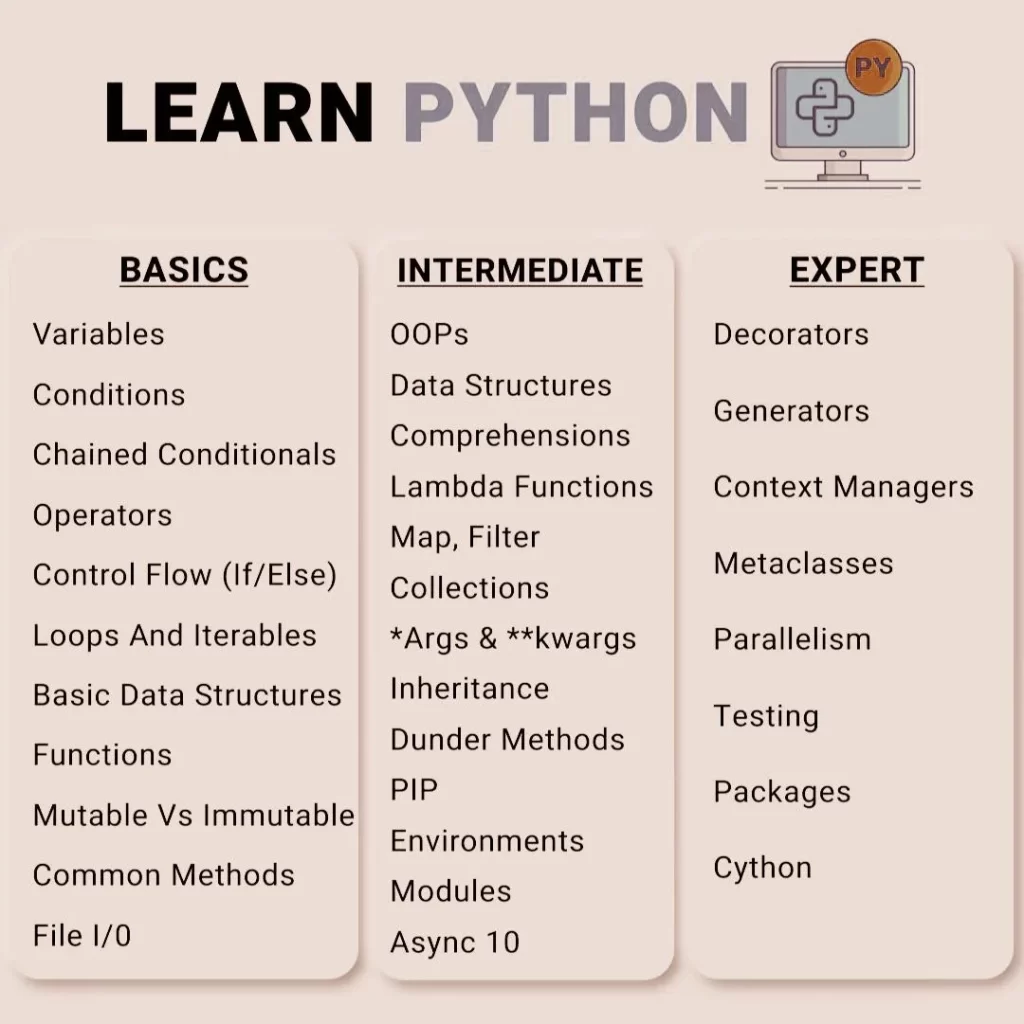
Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm that uses objects and classes to organize code. Python supports OOP, allowing you to create classes, define methods, and instantiate objects.
Modules and Libraries
Python’s extensive library ecosystem makes extending the language’s capabilities easy. Modules are files containing Python code that can be imported into other programs, while libraries are collections of modules. Some popular libraries include NumPy for numerical computing, Pandas for data analysis, and Matplotlib for data visualization.
Useful Python Tools
Python offers a variety of tools and resources to enhance your programming experience.
Python Compiler
A Python compiler translates Python code into machine code that a computer can execute. Popular Python compilers include CPython and PyPy.
Integrated Development Environments (IDEs)
IDEs provide a comprehensive platform for writing and testing Python code. Some popular IDEs include PyCharm, VS Code, and Jupyter Notebook.
Real-World Python Applications
Python is used in various applications, from web development to data analysis. Here are some examples:
- Web Development: Python frameworks like Django and Flask are used to build dynamic web applications.
- Data Analysis: Libraries like Pandas and Matplotlib are used to analyze and visualize data.
- Machine Learning: Libraries like Scikit-learn and TensorFlow are used to build machine learning models.
- Automation: Python scripts are used to automate repetitive tasks and improve efficiency.
FAQs
What is Python?
Python is a high-level programming language known for its readability and versatility. It is widely used for web development, data analysis, machine learning, and more.
How can I learn Python?
You can learn Python through online courses, tutorials, and books. Practice is key, so work on projects and exercises to improve your skills.
What are some popular Python libraries?
Popular Python libraries include NumPy, Pandas, Matplotlib, Scikit-learn, and TensorFlow. These libraries provide tools for data analysis, visualization, machine learning, and more.
What is a Python dictionary?
A Python dictionary is a collection of key-value pairs that allows you to store and access data using unique keys.